ARM Programming
Here you can find different articles about programming arm processors. I mainly work with cortex m3 design implemented by NXP on the evaluationboard by microbuilder.eu.
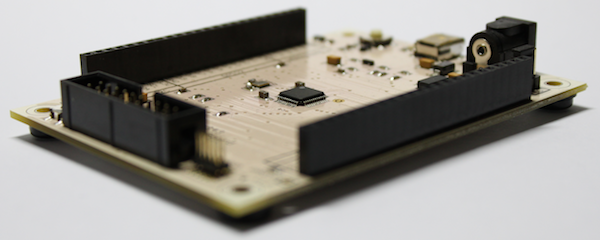
Ever if there is some problem i had do do more research to solve it, i will write it down here.
Linux Development Environment for the LPC1343 Reference Board by microBuilder
For ubuntu the gcc-arm-none-eabi toolchain is now in the standard repositories and can be installed via
sudo apt install gcc-arm-none-eabiNow for the LPC1343 Reference Board by microBuilder.eu there is a github repository that you should check out:
git clone https://github.com/microbuilder/LPC1343CodeBase.gitIn this repository you can find various examples. To activate each example you simply copy the according main.c file from tools/examples/ to the main repository directory and after some changes in the projectconfig.c you're half ready to compile the firmware successfully. The only thing missing for the linux environment is the tool to build the crc sum for the firmware. Without that you may be able to compile and even transfer the firmware to your board but it will not execute as it is rejected by the bootloader on transfer because it's lacking a checksum.
That's why you need either to copy it or compile and copy it:
cp tools/lpcrc/bin/lpcrc-linux . && chmod u+x lpcrc-linuxor
cd tools/lpcrc/ make cd ../../ && cp tools/lpcrc/lpcrc .Now you can compile and transfer your program defined as per chosen main.c.
Toolchain installation - Linux
Meanwhile the toolchain installation is much more easy for most common linux distributions: it's in the repo and can be installed from there.
The following is kept on this site only for documentary purposes.
To setup the toolchain for cortex-m3 microcontroller under linux there exists a gnu-arm-installer package from Waipot Ngamsaad.
To install the toolchain with this script there are some packages that need to be installed first.
apt-get install patch texinfo libncurses5-dev libx11-dev libgmp3-dev libmpfr-dev cd ~ mkdir arm-none-eabi cd arm-none-eabi svn checkout http://hobbycode.googlecode.com/svn/trunk/gnu-arm-installer gnu-arm-installer cd gnu-arm-installer/src/ wget http://ftp.gnu.org/gnu/binutils/binutils-2.19.tar.bz2 wget http://ftp.gnu.org/gnu/gcc/gcc-4.3.2/gcc-4.3.2.tar.bz2 wget ftp://ftp.fi.debian.org/pub/gentoo/distfiles/newlib-1.16.0.tar.gz wget ftp://gcc.gnu.org/pub/insight/releases/insight-6.8-1.tar.bz2 cd ..
Gnuarm (which is used heavily used by the script of Waipod Ngamsaad) is not always on and therefore you should search for some package-mirrors for the packages and download the packages there.
As insight-6.8.tar.bz2 is not the most recent version - respectively it has got some bigger errors - insight-6.8-1.tar.bz2 has been downloaded. To use this we have to make some changes in the gnu-arm-installer.sh:
INSIGHT_SRC=insight-6.8-1.tar.bz2 INSIGHT_VERSION=6.8-1 INSIGHT_DIR=insight-$INSIGHT_VERSION
It is a matter of taste to use the toolchain in form of "arm-elf" or "arm-none-eabi" (some people tell that "elf" is the old name for "eabi", others that "elf" requires an operation system... As they have to produce binaries for the cortex it is regardeless of which name it is given).
As existing Makefiles (for example for the source-codes for cortex m3 from microbuilder.eu) use arm-none-eabi i decided to %s/arm-elf/arm-none-eabi/g in gnu-arm-installer.sh (an other approach would be to have both by using symbolic links for the binries).
Whatever way you decide to go - the toolchain can now be setup with:
./gnu-arm-installer.sh
Now the install/bin-directory has to be put into PATH:
vim ~/.bashrc
input:
export PATH=~/arm-none-eabi/install/bin/:$PATH
and there you go with your cortex m3 and many other arm-platforms.
Toolchain installation - Mac OS X
To program OpenBeacon Tags from Bitmanufaktur for example (as they are shipped with a cortex m3 mircocontroller) or the above seen evaluation board by microbuider.eu you need a toolchain to compile your sourcecode.
The Script below is for setting up the toolchain on Mac OS X.
Before you run the script it is required to install gmp as well as mprf for example via MacPorts:
sudo port install gmp mpfr
Now you can setup your toolchain with this script:
#!/bin/sh # # Copyright (c) 2008 the NxOS developers # # See AUTHORS for a full list of the developers. # # Redistribution of this file is permitted under # the terms of the GNU Public License (GPL) version 2. # # Build an ARM cross-compiler toolchain (including binutils, gcc and # newlib) on autopilot. ROOT=`pwd` SRCDIR=$ROOT/src BUILDDIR=$ROOT/build PREFIX=$ROOT/install GCC_URL=http://ftp.gnu.org/pub/gnu/gcc/gcc-4.4.0/gcc-core-4.4.0.tar.bz2 GCC_VERSION=4.4.0 GCC_DIR=gcc-$GCC_VERSION BINUTILS_URL=http://ftp.gnu.org/gnu/binutils/binutils-2.19.1.tar.bz2 BINUTILS_VERSION=2.19.1 BINUTILS_DIR=binutils-$BINUTILS_VERSION NEWLIB_URL=ftp://sources.redhat.com/pub/newlib/newlib-1.17.0.tar.gz NEWLIB_VERSION=1.17.0 NEWLIB_DIR=newlib-$NEWLIB_VERSION GDB_URL=ftp://ftp.gnu.org/gnu/gdb/gdb-6.8a.tar.bz2 GDB_VERSION=6.8 GDB_DIR=gdb-$GDB_VERSION echo "I will build an arm-elf cross-compiler: Prefix: $PREFIX Sources: $SRCDIR Build files: $BUILDDIR Software: Binutils $BINUTILS_VERSION Gcc $GCC_VERSION Newlib $NEWLIB_VERSION Gdb $GDB_VERSION Press ^C now if you do NOT want to do this." read IGNORE # # Helper functions. # ensure_source() { URL=$1 FILE=$(basename $1) if [ ! -e $FILE ]; then echo "getting $FILE" curl -L -O$FILE $URL fi } unpack_source() { ( cd $SRCDIR ARCHIVE_SUFFIX=${1##*.} if [ "$ARCHIVE_SUFFIX" = "gz" ]; then tar zxvf $1 elif [ "$ARCHIVE_SUFFIX" = "bz2" ]; then tar jxvf $1 else echo "Unknown archive format for $1" exit 1 fi ) } # Create all the directories we need. mkdir -p $SRCDIR $BUILDDIR $PREFIX ( cd $SRCDIR # First grab all the source files... ensure_source $GCC_URL ensure_source $BINUTILS_URL ensure_source $NEWLIB_URL #rboissat: Adding GNU gdb ensure_source $GDB_URL # ... And unpack the sources. unpack_source $(basename $GCC_URL) unpack_source $(basename $BINUTILS_URL) unpack_source $(basename $NEWLIB_URL) unpack_source $(basename $GDB_URL) ) # Set the PATH to include the binaries we're going to build. OLD_PATH=$PATH export PATH=$PREFIX/bin:$PATH # # Stage 1: Build binutils # ( mkdir -p $BUILDDIR/$BINUTILS_DIR cd $BUILDDIR/$BINUTILS_DIR $SRCDIR/$BINUTILS_DIR/configure --target=arm-elf --prefix=$PREFIX \ --disable-werror --enable-interwork --enable-multilib --with-float=soft \ && make all install ) || exit 1 # # Stage 2: Patch the GCC multilib rules, then build the gcc compiler only # ( MULTILIB_CONFIG=$SRCDIR/$GCC_DIR/gcc/config/arm/t-arm-elf echo " MULTILIB_OPTIONS += mno-thumb-interwork/mthumb-interwork MULTILIB_DIRNAMES += normal interwork " >> $MULTILIB_CONFIG mkdir -p $BUILDDIR/$GCC_DIR cd $BUILDDIR/$GCC_DIR $SRCDIR/$GCC_DIR/configure --target=arm-elf --prefix=$PREFIX \ --enable-interwork --enable-multilib --with-float=soft \ --with-libiconv-prefix=/opt/local \ --with-gmp-include=/opt/local/include --with-mpfr-include=/opt/local/include \ --with-gmp-lib=/opt/local/lib --with-mpfr-lib=/opt/local/lib \ --enable-languages="c" --with-newlib \ --with-headers=$SRCDIR/$NEWLIB_DIR/newlib/libc/include \ && make all-gcc install-gcc ) || exit 1 # # Stage 3: Build and install newlib # ( # And now we can build it. mkdir -p $BUILDDIR/$NEWLIB_DIR cd $BUILDDIR/$NEWLIB_DIR $SRCDIR/$NEWLIB_DIR/configure --target=arm-elf --prefix=$PREFIX \ --enable-interwork --enable-multilib --with-float=soft \ && make all install ) || exit 1 # # Stage 4: Build and install the rest of GCC. # ( cd $BUILDDIR/$GCC_DIR make all install ) || exit 1 # # Stage 5: Build and install GDB # ( mkdir -p $BUILDDIR/$GDB_DIR cd $BUILDDIR/$GDB_DIR $SRCDIR/$GDB_DIR/configure --target=arm-elf --prefix=$PREFIX \ --disable-werror --enable-interwork --enable-multilib --with-float=soft \ && make all install ) || exit 1 export PATH=$OLD_PATH echo "export PATH=$PREFIX/bin:\$PATH">$ROOT/env.sh echo " Build complete! To use your new toolchain: - Source the $ROOT/env.sh script in your shell. In bash: source $ROOT/env.sh - Or, just add $PREFIX/bin to your PATH manually. "